Zeenea GraphQL Catalog API
Welcome to the Zeenea GraphQL Catalog API reference!
API Endpoints
https://<your_tenant>.zeenea.app/api/catalog/graphql
Headers
X-API-SECRET: <YOUR_API_SECRET_HERE>
Introduction
This reference includes the complete set of GraphQL types, queries, mutations, and their parameters for retrieving catalog items and mutating them (updates, deletes and creates).
Authentication
To use the API, you must provide a valid API key in the HTTP headers of your requests.
Here are the steps to authenticate your calls:
- Create a new API Key in the dedicated section of the Admin page (https://your_tenant.zeenea.app/admin/settings/api-keys)
- Make sure to store the API SECRET in a secure location, as it will no longer be displayed after you close the window
- In your HTTP requests, add the following headers:
- X-API-SECRET: {api-secret-value}
GraphQL schema
There are several ways to retrieve the GraphQL schema definition (that can be necessary to generate client code). First, you can leverage GraphQL introspection APIs:
With Gradle/Apollo plugin
./gradlew downloadApolloSchema --endpoint="https://_your_tenant_.zeenea.app/api/catalog/graphql" \
--schema=schema.graphql --header="X-API-SECRET: TOKEN_API_SECRET_HERE"
With Python/sgqlc
py -m sgqlc.introspection -H "X-API-SECRET: TOKEN_API_SECRET" -H "Accept: application/json" \
https://_your_tenant_.zeenea.app/api/catalog/graphql schema.graphql
Or you can just download the latest schema here
Principles
Zeenea Catalog API leverages GraphQL to allow querying the catalog graph while defining precisely the properties and edges that you need in the result.
Zeenea Catalog API is based on GraphQL. To learn more about GraphQL, and how it differs from traditional REST APIs, please visit the official GraphQL website.
Zeenea Catalog API also follows Relay convention. While Relay has initially been designed to support React web development at scale on top of GraphQL, it is also a very opiniated framework on how to design a GraphQL API, and especially how to manage connections between entities in a GraphQL schema. Following those conventions allows developers to use one of the Relay client libraries to simplify script development, especially handling paginated lists of connected items.
You can learn more on Relay on the official Relay website.
As an example is often more explanatory than long sentences, here is a query that will retrieve a dataset with a couple of properties, and two connections: fields and curator.
$ref = 'DATABASE/starwars/rockets';
query datasetsAndFields($ref: ItemReference!) {
item(ref: $ref) {
type
key
name
schema: property(ref: "schema")
table_name: property(ref: "source_name")
classification: property(ref: "classification")
domain: property(ref: "business_domain")
fields: connection(ref: "fields") {
nodes {
name
type
pii: property(ref: "PII")
}
}
curators: connection(ref: "curators", first: 1) {
nodes {
name
email: property(ref: "email")
}
}
}
}
This illustrates the main principles of the API:
- items are located using a reference, that can carry different ways to identify an object
- properties defined in the metamodel can be retrieved by using a reference (eg.
classification
), and theproperty
pseudo-field defined in the graphql schema - lists of connected items can be retrieved by using a named alias (eg.
fields
), and theconnection
pseudo-field defined in the graphql schema
The former query would produce the following payload:
{
"data": {
"type": "dataset",
"key": "DATABASE/starwars/rockets",
"name": "Star wars rockets",
"schema": "starwars",
"table_name": "rockets",
"classification": "Internal",
"domain": "Space exploration",
"fields": {
"nodes": [
{
"name": "id",
"type": "Number",
"pii": "false"
},
{
"name": "model",
"type": "Text",
"pii": "false"
},
{
"name": "pilot",
"type": "Text",
"pii": "true"
}
]
},
"curators": {
"nodes": [
{
"name": "Paul Smith",
"email": "psmith@nowhere.far"
}
]
}
}
}
Using the API requires former knowledge of the metamodel defined in the target catalog (item types, properties, links).
Generic item model
Unlike most GraphQL APIs, Zeenea relies on a very generic GraphQL schema that reflects the very dynamic nature of the Zeenea Metamodel.
The GraphQL schema exposed by the API is the following:
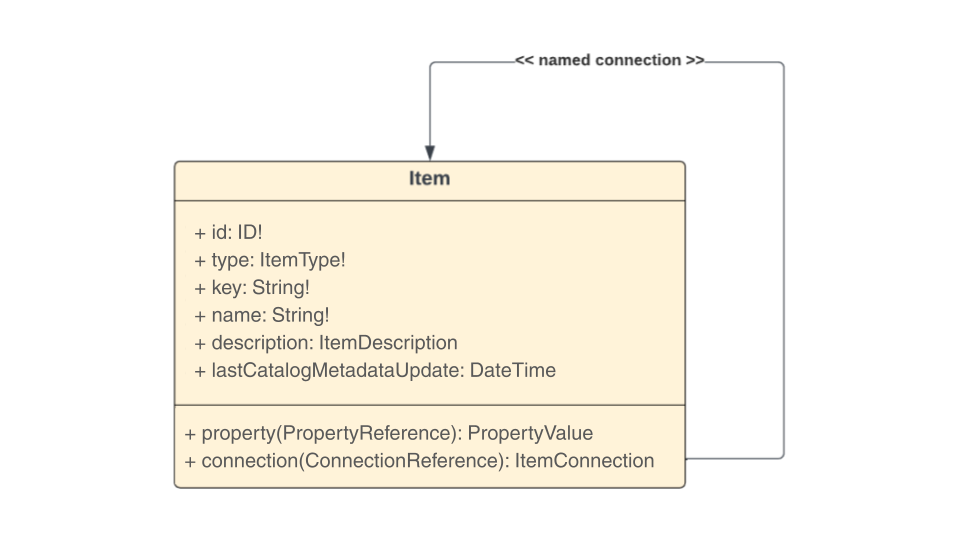
The schema mostly relies on a single type, Item, that contains a few attributes that are common to all item types, and two pseudo-fields :
property
, used to retrieve the value or values of a property defined in the metamodelconnection
, used to retrieve a collection of items linked to their parent through a named link.
In Zeenea's data model, all links between items are bi-directional and may have different cardinalities. In the GraphQL API, links are fetched in a given direction (from a parent item), using the name of the relation in that direction, and are always considered having a many cardinality.
For instance, datasets
and fields
are linked in the Zeenea model by a one-to-many relationship. In the GraphQL API, it will map to 2 differents named connections:
- from the dataset, you can fetch the
fields
connection to get the list of all the items of type field of the dataset - from the field, you can fetch the
dataset
connection to fetch the item of type dataset. It will be mapped to a connection of size 1.
Properties and connections are identified by codes. Those codes are defined in the metamodel.
Item is an interface, that is specialized for some of the item types to provide information that is specific to that type. So far, only the Dataset and Data Process type is specialized.
You can retrieve type-specific information by using the ...on
GraphQL operator. A sample query illustrates its use in the Examples section below.
Reference system
To retrieve information, the API relies on a set of reference types to identify items or elements of the metamodel.
The ItemReference is used to reference an item. It can map to the item unique ID (retrieved from the API) or one of the unique keys available for an item.
The PropertyReference is the way to retrieve a property of an item. It maps to the code or the name of the property (case sensitive) as defined in the metamodel. Properties can be user-defined (then the name is available in the user-defined metamodel), or built-in.
Built-in property are referenced here.
The ConnectionReference identifies links between items. Links are bi-directional by design, but may have different names depending on the direction used to fetch them.
Connection names are referenced here.
Using connections
Similarly to properties, connections are identified by code using the ConnectionReference type.
As connections can be of any size, they are always paginated, using the standard Relay mechanism. The default (and max) page size is 20.
To iterate through a connection pages, you must use the PageInfo object. See sample here.
Dealing with errors
Except when there is a server or access failure (that will raise a 40X or 5XX HTTP response code), GraphQL always returns a HTTP Status Code 200, and potential errors are included in the response payload.
The generic structure of a GraphQL query is the following (see GraphQL spec for a comprehensive documentation).
{
"errors": [ { ... }],
"data": { ... },
"extensions" : { ... }
}
An error object has the following form (we use the extensions
mechanism to provide more context to the error, especially when the error comes from reference resolution):
{
"message": "Property domain has not been found",
"path": ["contact", "domain"],
"locations": [
{
"line": 6,
"column": 3
}
],
"extensions": {
"code": "PROPERTY_NOT_FOUND",
"value": "domain",
"availableValues": ["firstName", "lastName", "email", "phone"]
}
}
The list of error codes can be found here
Complexity check
GraphQL provides a very powerful semantic to query a graph-based system. This power comes with a drawback: it is theoretically possible to forge a query that would retrieve the entire graph, or recursively load connections with an infinite depth - leading to potentially disastrous performance issues.
To protect the system from over complex queries, Zeenea performs a complexity check on every query. Complexity check is performed on the structure of the query, not on the size of the results - this means that a query that passes the check will always pass it, even if the size of the results increases.
The complexity score of a query is provided in the extensions
attribute of the GraphQL response payload:
{
"errors": [],
"data": {},
"extensions": {
"complexityScore": 20
}
}
If one of your query is too complex, you will have to decompose it to simpler sub-queries.
For instance, let's consider the following query:
query datasetsAndFields($datasetRef: ItemReference!) {
item(ref: $datasetRef) {
fields: connection(ref: "fields") {
nodes {
definitions: connection(ref: "definitions") {
nodes {
implementations: connection(ref: "implementations") {
nodes {
type
name
description
}
}
}
}
}
}
}
}
Submitting this query might raise a complexity check error (QUERY_TOO_COMPLEX
). In this case, you can reduce the depth of the initial query by removing one of the connection traversals:
query datasetsAndFields($datasetRef: ItemReference!) {
item(ref: $datasetRef) {
fields: connection(ref: "fields") {
nodes {
definitions: connection(ref: "definitions") {
nodes {
key
}
}
}
}
}
}
While iterating the definitions
connection, you can submit a sub-query to retrieve the implementations
connection, using the key of the parent item. For each item in the definitions
connection, you can run the sub-query:
query businessTermImplementations($key: ItemReference!) {
connection(sourceRef: $key, connectionRef: "implementations") {
nodes {
type
name
description
}
}
}
Naming reference
Item types
Item type | Value |
---|---|
Dataset | dataset |
Field | field |
Visualization | visualization |
Data process | data-process |
Contact | contact |
Datasource | datasource |
Category | category |
Custom Item Type | Code of the custom item type as defined in Zeenea metamodel |
Glossary Item Type | Code of the glossary item type as defined in Zeenea metamodel |
Built-in properties
The following table is the reference for all built-in properties:
Property | Type | Available on | Read/write | Description |
---|---|---|---|---|
sourceName | String | Dataset, Field, Visualization, DataProcess | Read-only | Name of the item in the source system |
sourceDescription | String | Dataset, Field, Visualization, DataProcess | Read-only | Description of the item in the source system |
lastSourceMetadataUpdate | Date | Dataset, Field, Visualization, DataProcess | Read-only | Last time there was a change in the item metadata in the source system |
orphan | Boolean | Dataset, Field | Read-only | Item is missing from the last inventory |
deletionDate | Date | Dataset, Field, Visualization, DataProcess | Read-only | The date at which the item has been deleted in the source system |
importDate | Date | Dataset, Field, Visualization, DataProcess | Read-only | The date at which the item has been imported into the catalog |
fieldType | String | Field | Read-only | The normalized type of the field |
fieldNativeType | String | Field | Read-only | The native type of the field as defined in the source system |
canBeNull | Boolean | Field | Read-only | Whether the field is nullable |
multivalued | Boolean | Field | Read-only | Whether the field supports multiple values |
primaryKey | Boolean | Field | Read-only | Whether the field is a primary key |
foreignKey | Boolean | Field | Read-only | Whether the field is a foreign key |
businessKey | Boolean | Field | Read-write | Whether the field is a business key |
dataProfileEnabled | Boolean | Field | Read-write | Whether the data profile on the field is enabled |
dataProfilePublished | Boolean | Field | Read-write | Whether the data profile on the field is published |
alternativeNames | [String] | All glossary types | Read-write | List of alternatives names |
String | Contact | Read-write | Email of the contact | |
firstName | String | Contact | Read-write | First name of the contact |
lastName | String | Contact | Read-write | Last name of the contact |
phone | String | Contact | Read-write | Phone number of the contact |
Connections
The following table is the reference for all available connections between items:
Source type | Connection name | Target type(s) | Read/write | Cardinal | Description |
---|---|---|---|---|---|
dataset |
fields |
field |
Read-only | 1-n | Fields of a dataset |
dataset |
relations |
dataset |
Read-only | 0-n | Datasets linked through a foreign key |
dataset |
ingesters |
data-process |
Read-write | 0-n | All the data processes that have the dataset as input |
dataset |
producers |
data-process |
0-n | 0-n | All the data processes that have the dataset as output |
dataset |
visualization |
visualization |
0-1 | 0-n | For the datasets that are embedded in a visualisation, the visualization |
dataset |
Custom item type name | Custom item type | 0-n | 0-n | Custom items of a given type linked to the dataset |
dataset |
category |
category |
0-1 | 0-n | [DEPRECATED] Category of the dataset |
dataset |
curators |
contact |
0-n | 0-n | Curators of the dataset |
dataset |
Responsibility name | contact |
0-n | 0-n | Contacts that have the given responsibility on the dataset |
dataset |
datasource |
datasource |
1-1 | 0-n | The datasource of the dataset |
dataset |
definitions |
All glossary item types | 0-n | 0-n | All the glossary items linked to the dataset (can contain various types) |
field |
dataset |
dataset |
1-1 | 0-n | The dataset the field belongs to |
field |
Custom item type name | Custom item type | 0-n | 0-n | Custom items of a given type linked to the field |
field |
definitions |
All glossary item types | 0-n | 0-n | All the glossary items linked to the field (can contain various types) |
field |
curators |
contact |
0-n | 0-n | Curators of the field |
field |
Responsibility name | contact |
0-n | 0-n | Contacts that have the given responsibility on the field |
data-process |
inputs |
dataset or Custom item type |
0-n | 0-n | Inputs of the data process |
data-process |
outputs |
dataset , visualization or Custom item type |
0-n | 0-n | Outputs of the data process |
data-process |
Custom item type name | Custom item type | 0-n | 0-n | Custom items of a given type linked to the data process |
data-process |
curators |
contact |
0-n | 0-n | Curators of the data process |
data-process |
Responsibility name | contact |
0-n | 0-n | Contacts that have the given responsibility on the data process |
data-process |
datasource |
datasource |
0-1 | 0-n | The datasource of the data process (if teh data process has been harvested) |
data-process |
definitions |
All glossary item types | 0-n | 0-n | All the glossary items linked to the data process (can contain various types) |
visualization |
Custom item type name | Custom item type | 0-n | 0-n | Custom items of a given type linked to the data process |
visualization |
datasets |
dataset |
0-n | 0-n | Datasets embedded in the visualization |
visualization |
curators |
contact |
0-n | 0-n | Curators of the data process |
visualization |
Responsibility name | contact |
0-n | 0-n | Contacts that have the given responsibility on the visualization |
visualization |
datasource |
datasource |
1-1 | 0-n | The datasource of the visualization |
visualization |
definitions |
All glossary item types | 0-n | 0-n | All the glossary items linked to the visualization (can contain various types) |
Custom item type | members |
Any type | 0-n | 0-n | All the other items that are linked to this custom item |
Custom item type | ingesters |
data-process |
0-n | 0-n | All the data processes that have the custom item as input |
Custom item type | producers |
data-process |
0-n | 0-n | All the data processes that have the custom item as output |
Custom item type | curators |
contact |
0-n | 0-n | Curators of the custom item |
Custom item type | Responsibility name | contact |
0-n | 0-n | Contacts that have the given responsibility on the custom item |
Custom item type | definitions |
All glossary item type | 0-n | 0-n | All the glossary items linked to the custom item (can contain various types) |
Glossary item type | implementations |
Any type | 0-n | 0-n | All the other items that are linked to the glossary item |
Glossary item type | parents |
All glossary item type | 0-n | 0-n | Parents of the glossary item in the glossary |
Glossary item type | children |
All glossary item type | 0-n | 0-n | Children of the glossary item in the glossary |
Glossary item type | curators |
contact |
0-n | 0-n | Curators of the glossary item |
Glossary item type | Responsibility name | contact |
0-n | 0-n | Contacts that have the given responsibility on the glossary item |
contact |
curator |
Any type | 0-n | 0-n | All the items the contact is curator of (various types) |
contact |
Responsibility name | Any type | 0-n | 0-n | All the items on which the contact has the given responsibility(various types) |
datasource |
imports |
dataset , field , visualization , data-process |
0-n | 0-n | All the items that have been imported from the source |
category |
members |
dataset |
0-n | 0-n | [DEPRECATED] Datasets of the category |
category |
curators |
contact |
0-n | 0-n | [DEPRECATED] Curators of the custom item |
category |
Responsibility name | contact |
0-n | 0-n | [DEPRECATED] Contacts that have the given responsibility on the custom item |
category |
definitions |
All glossary item type | 0-n | 0-n | [DEPRECATED] All the glossary items linked to the category (can contain various types) |
Error codes
Error Code | Description | Extensions |
---|---|---|
ITEM_NOT_FOUND |
When an item does not match any id, key, name, source name with the reference. | code, value |
TOO_MANY_ITEMS_FOUND |
When multiple items have the same name or sourceName | code, value |
TOO_MANY_ITEMS_FOUND_WITH_TYPE |
When multiple items have the same name with the item type given in parameters | code, value |
MISSING_ARGUMENT |
When an argument from a field or a direct query is not provided | code, field, arguments |
QUERY_TOO_COMPLEX |
When the submitted query has a complexity score above the max allowed score | code, complexityScore, maxAllowedScore |
ITEM_TYPE_NOT_FOUND |
When the argument type of type ItemType does not correspond to an existing value. All available values are provided in the corresponding extension field | code, value, availableValues |
CONNECTION_REFERENCE_NOT_FOUND |
When the argument ref of type ConnectionReference does not correspond to an existing value. All available values are provided in the corresponding extension field | code, value, availableValues |
PROPERTY_NOT_FOUND |
When a property reference does not correspond to an existing value. All available values are provided in the corresponding extension field | code, value, availableValues |
TOO_MANY_PROPERTIES_FOUND |
When multiple properties have been found with the same name | code, value, |
PROPERTY_VALUE_INVALID |
When the user has submitted a value for a property mutation command that does not fit the format of the property | code, propertyRef, value, propertyType, availableValues |
COMMAND_NOT_FOUND |
When a mutation command refers to a non existing command. | code, value, availableValues |
COMMAND_INVALID |
When a mutation command cannot be applied to the current value. | code, value, currentValue |
COMMAND_UNAVAILABLE |
When a mutation command cannot be applied to the type of property | code, value, propertyType, availableValues |
ITEM_TYPE_INVALID |
When the pair connection reference / item type provided in a mutation does not correspond to any connection | code, value, availableValues |
ITEM_TYPE_INVALID |
When a create item request is used for a non autorized type (Dataset, Field, Visualization) | code, value, availableValues |
ITEM_TYPE_INVALID |
When a delete item request attempt to delete a datasource | code, value, availableValues |
COMMAND_UNAVAILABLE |
When a mutation command is not available for the edge mutation | code, value, availableValues |
CONNECTION_VALUE_INVALID |
When an edge mutation command tries to edge a contact as a curator, without having a user associated to the contact | code, value |
READ_ONLY_PROPERTY |
When a create or update item request attempt to set a built-in property or attempt to set a read-only property | code, propertyRef |
MISSING_MANDATORY_FIELD |
When a create item request lacks a mandatory field (item type or name) | code, arguments |
TOO_MANY_ITEMS_FOUND_WITH_KEY |
When a createItem or updateItem request uses a key already used by another item in the catalog | code, value |
ITEM_INVALID |
When a deleteContact request attempt to delete a contact associated to a user | code, value |
TOO_MANY_ITEMS_FOUND_WITH_EMAIL |
When a createContact request attempt to create a contact with an already used email | code, value |
*ATTRIBUTE*_VALUE_INVALID |
When a createContact, updateContact, createItem, updateItem request attempt to create or update a contact or item with an invalid value for a basic attribute (too long name, email without @, etc.) | code, value |
READ_ONLY_CONTACT_EMAIL |
When an updateContact request attempt to modify the email of a user | code, value |
CONNECTION_REFERENCE_UNAVAILABLE |
When a createContact request attempt to create a curator connection while there is no associated user | code, value, availableValues |
PREDICATE_UNAVAILABLE |
When an items request uses a predicate that can not be applied to the type of property | code, propertyRef, value, propertyType, availableValues |
FILTER_VALUE_INVALID_FOR_PREDICATE |
When an items request uses a property value that is invalid for the used predicate | code, value |
FILTER_VALUE_INVALID |
When the user has submitted a value for a filter that does not fit the format of the property | code, propertyRef, value, propertyType, availableValues |
ITEM_TYPE_NOT_IMPLEMENTED |
When an items request attempts to retrieve items for an item type that is not implemented for this query yet | code, value |
PROPERTY_NOT_IMPLEMENTED |
When a query or mutation attempts to use a property reference that is not implemented yet | code, value |
FILTER_TYPE_NOT_IMPLEMENTED |
When an items request attempts to use a filter type that is not implemented yet | code, value |
Examples
The following section provides various samples for common queries.
Retrieve all fields of a dataset and its datasource
$ref = 'DATABASE/schema/table_name';
query datasetsAndFields($ref: ItemReference!) {
item(ref: $ref) {
type
key
name
schema: property(ref: "schema")
table_name: property(ref: "source_name")
datasource: connection(ref: "datasource") {
nodes {
name
}
}
fields: connection(ref: "fields") {
nodes {
name
type
pii: property(ref: "PII")
}
}
}
}
Retrieve data quality statement for a dataset
To cast an Item to one of its specialization, you must use the ...on
GraphQL operator:
$ref = 'DATABASE/schema/table_name';
query datasetQuality($ref: ItemReference!) {
item(ref: $ref) {
... on Dataset {
name
schema: property(ref: "schema")
table_name: property(ref: "source_name")
quality {
syntheticResult
checks {
name
family
result
}
}
}
}
}
Retrieve all datasets owned by a given user
$ref = 'john.doe@nowhere.far';
query ownership($ref: ItemReference!) {
item(ref: $ref) {
type
key
email: property(ref: "email")
curators: connection(ref: "curator", filter: { nodeTypes: ["dataset"] }) {
nodes {
schema: property(ref: "schema")
table_name: property(ref: "source_name")
datasource: connection(ref: "datasource") {
nodes {
name
}
}
}
}
}
}
Iterate a connection
$ref = 'DATABASE/schema/table_name';
query datasetsAndFields($ref: ItemReference!) {
item(ref: $ref) {
type
key
name
schema: property(ref: "schema")
table_name: property(ref: "source_name")
fields: connection(ref: "fields") {
pageInfo {
hasNextPage
endCursor
}
nodes {
name
type
pii: property(ref: "PII")
}
}
}
}
Sub-sequent queries (if item.fields.pageInfo.hasNextPage
):
$ref = item.key;
$endCursor = item.fields.pageInfo.endCursor;
query fieldsNextPage($ref: ItemReference!, $endCursor: String) {
connection(sourceRef: $ref, connectionRef: "fields", after: $endCursor) {
pageInfo {
hasNextPage
endCursor
}
nodes {
name
type
pii: property(ref: "PII")
}
}
}
Create a new custom item
mutation createApplication {
createItem(
input: {
type: "application"
name: "The Application"
properties: [
{ ref: "business_domains", value: ["Finance", "Controlling"] }
{ ref: "criticality", value: ["Business critical"] }
]
connections: [
{ connectionRef: "curators", itemRefs: ["john.doe@nowhere.far"] }
{
connectionRef: "members"
itemRefs: ["741ae65e-9db2-4b5e-b0c4-265abf51f009", "05050ac8-fad1-4412-862e-020515b33c40"]
}
]
}
) {
item {
id
}
}
}
Change a dataset curator
$ref = 'DATABASE/schema/table_name';
$curator = 'john.doe@nowhere.far';
mutation updateDatasetCurator($ref: ItemReference!, $curator: ItemReference) {
updateItem(
input: {
ref: $ref
updates: { connections: [{ command: REPLACE, connectionRef: "curators", itemRefs: [$curator] }] }
}
) {
item {
id
}
}
}
Queries
glossary
Description
Query a list of semantic items from the catalog.
Response
Returns an ItemConnection!
Example
Query
query Glossary(
$after: String,
$before: String,
$first: Int,
$last: Int
) {
glossary(
after: $after,
before: $before,
first: $first,
last: $last
) {
nodes {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
edges {
cursor
node {
...ItemFragment
}
}
pageInfo {
startCursor
hasNextPage
hasPreviousPage
endCursor
}
totalCount
}
}
Variables
{
"after": "abc123",
"before": "xyz789",
"first": 987,
"last": 987
}
Response
{
"data": {
"glossary": {
"nodes": [Item],
"edges": [ItemEdge],
"pageInfo": PageInfo,
"totalCount": 123
}
}
}
item
Description
Query one item from the catalog, using a unique reference.
Response
Returns an Item!
Arguments
Name | Description |
---|---|
ref - ItemReference!
|
Reference used to identify the item |
Example
Query
query Item($ref: ItemReference!) {
item(ref: $ref) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
}
}
Variables
{"ref": "DATABASE/schema/table_name"}
Response
{
"data": {
"item": {
"id": "4",
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789"
}
}
}
itemByName
Description
Query one item from the catalog, using its name and type.
Response
Returns an Item!
Example
Query
query ItemByName(
$name: String!,
$type: ItemType!
) {
itemByName(
name: $name,
type: $type
) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
}
}
Variables
{
"name": "abc123",
"type": "dataset"
}
Response
{
"data": {
"itemByName": {
"id": 4,
"type": "dataset",
"key": "abc123",
"name": "abc123",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789"
}
}
}
items
Description
Query a list of items of a given type from the catalog.
Response
Returns an ItemConnection!
Arguments
Name | Description |
---|---|
type - ItemType!
|
The type of the items to fetch |
filters - [ItemFilterInput!]
|
A list of filters (predicates) to narrow the list. Filters are combined with the AND operator |
after - String
|
Used for pagination. Should be set to the 'endCursor' value of a connection PageInfo |
before - String
|
NOT SUPPORTED (only forward pagination is supported) |
first - Int
|
Amount of items to query |
last - Int
|
NOT SUPPORTED (only forward pagination is supported) |
Example
Query
query Items(
$type: ItemType!,
$filters: [ItemFilterInput!],
$after: String,
$before: String,
$first: Int,
$last: Int
) {
items(
type: $type,
filters: $filters,
after: $after,
before: $before,
first: $first,
last: $last
) {
nodes {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
edges {
cursor
node {
...ItemFragment
}
}
pageInfo {
startCursor
hasNextPage
hasPreviousPage
endCursor
}
totalCount
}
}
Variables
{
"type": "dataset",
"filters": [ItemFilterInput],
"after": "xyz789",
"before": "abc123",
"first": 123,
"last": 123
}
Response
{
"data": {
"items": {
"nodes": [Item],
"edges": [ItemEdge],
"pageInfo": PageInfo,
"totalCount": 123
}
}
}
node
Description
Direct Query to a Node (to follow Relay conventions).
Mutations
createContact
Description
Creates a contact.
Response
Returns a CreateItemPayload!
Arguments
Name | Description |
---|---|
input - CreateContactInput
|
List of input fields to create a contact |
Example
Query
mutation CreateContact($input: CreateContactInput) {
createContact(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
}
}
Variables
{"input": CreateContactInput}
Response
{
"data": {
"createContact": {
"clientMutationId": "abc123",
"item": Item
}
}
}
createContactV2
Description
Creates a contact.
Response
Returns a CreateItemPayloadV2!
Arguments
Name | Description |
---|---|
input - CreateContactInput
|
List of input fields to create a contact |
Example
Query
mutation CreateContactV2($input: CreateContactInput) {
createContactV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
}
}
Variables
{"input": CreateContactInput}
Response
{
"data": {
"createContactV2": {
"clientMutationId": "abc123",
"item": Item
}
}
}
createItem
Description
Creates an item. Available for Custom Items, Glossary Items, and Data Processes.
Response
Returns a CreateItemPayload!
Arguments
Name | Description |
---|---|
input - CreateItemInput
|
List of input fields to create an item |
Example
Query
mutation CreateItem($input: CreateItemInput) {
createItem(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
}
}
Variables
{
"input": {
"clientMutationId": "abc123",
"type": "data-process",
"key": "abc123",
"name": "abc123",
"description": {"content": "abc123"},
"properties": [{"ref": "domain", "value": ["Supply chain"]}],
"connections": [
{"connectionRef": "inputs", "itemRefs": ["DATABASE/schema/table_name"]}
]
}
}
Response
{
"data": {
"createItem": {
"clientMutationId": "xyz789",
"item": Item
}
}
}
createItemV2
Description
Creates an item. Available for Custom Items, Glossary Items, and Data Processes.
Response
Returns a CreateItemPayloadV2!
Arguments
Name | Description |
---|---|
input - CreateItemInput
|
List of input fields to create an item |
Example
Query
mutation CreateItemV2($input: CreateItemInput) {
createItemV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
}
}
Variables
{
"input": {
"clientMutationId": "abc123",
"type": "data-process",
"key": "abc123",
"name": "abc123",
"description": {"content": "abc123"},
"properties": [{"ref": "domain", "value": ["Supply chain"]}],
"connections": [
{"connectionRef": "inputs", "itemRefs": ["DATABASE/schema/table_name"]}
]
}
}
Response
{
"data": {
"createItemV2": {
"clientMutationId": "abc123",
"item": Item
}
}
}
deleteContact
Description
Deletes a contact.
Response
Returns a DeleteItemPayload!
Arguments
Name | Description |
---|---|
input - DeleteContactInput
|
List of input fields to delete a contact |
Example
Query
mutation DeleteContact($input: DeleteContactInput) {
deleteContact(input: $input) {
clientMutationId
}
}
Variables
{"input": DeleteContactInput}
Response
{
"data": {
"deleteContact": {
"clientMutationId": "xyz789"
}
}
}
deleteDataProcessOperations
Description
Delete operations list on Data Process
Response
Returns a DataProcess!
Arguments
Name | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Data Process |
Example
Query
mutation DeleteDataProcessOperations($ref: ItemReference!) {
deleteDataProcessOperations(ref: $ref) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
operations {
description {
...RichTextFragment
}
inputFields {
...FieldOperationFragment
}
outputFields {
...FieldOperationFragment
}
}
}
}
Variables
{"ref": "DATABASE/schema/table_name"}
Response
{
"data": {
"deleteDataProcessOperations": {
"id": "4",
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "abc123",
"operations": [DataProcessOperation]
}
}
}
deleteDataProcessOperationsV2
Description
Delete operations list on Data Process
Response
Returns an UpdateDataProcessPayload!
Arguments
Name | Description |
---|---|
input - DeleteDataProcessOperationsInput!
|
List of input fields to delete operations of a Data Process |
Example
Query
mutation DeleteDataProcessOperationsV2($input: DeleteDataProcessOperationsInput!) {
deleteDataProcessOperationsV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
operations {
...DataProcessOperationFragment
}
}
}
}
Variables
{"input": DeleteDataProcessOperationsInput}
Response
{
"data": {
"deleteDataProcessOperationsV2": {
"clientMutationId": "xyz789",
"item": DataProcess
}
}
}
deleteDataQualityStatement
Description
Deletes all Data Quality information of a Dataset.
Response
Returns a Dataset!
Arguments
Name | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Dataset |
Example
Query
mutation DeleteDataQualityStatement($ref: ItemReference!) {
deleteDataQualityStatement(ref: $ref) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
foreignKeys {
referencedDataset
links {
...RelationalLinkFragment
}
origin
}
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
quality {
originator
syntheticResult
trustScore
dashboardLink
checks {
...DataQualityCheckFragment
}
}
}
}
Variables
{"ref": "DATABASE/schema/table_name"}
Response
{
"data": {
"deleteDataQualityStatement": {
"id": 4,
"type": "dataset",
"key": "abc123",
"name": "abc123",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"foreignKeys": [ForeignKey],
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789",
"quality": DataQualityStatement
}
}
}
deleteDataQualityStatementV2
Description
Deletes all Data Quality information of a Dataset.
Response
Returns an UpdateDatasetPayload!
Arguments
Name | Description |
---|---|
input - DeleteDataQualityStatementInput!
|
List of input fields to delete data quality statement of a Dataset |
Example
Query
mutation DeleteDataQualityStatementV2($input: DeleteDataQualityStatementInput!) {
deleteDataQualityStatementV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
foreignKeys {
...ForeignKeyFragment
}
property
connection {
...ItemConnectionFragment
}
catalogCode
quality {
...DataQualityStatementFragment
}
}
}
}
Variables
{"input": DeleteDataQualityStatementInput}
Response
{
"data": {
"deleteDataQualityStatementV2": {
"clientMutationId": "abc123",
"item": Dataset
}
}
}
deleteForeignKeys
Description
Delete the foreign keys of a Dataset.
Foreign keys of Source
origin will be left untouched.
Response
Returns a Dataset!
Arguments
Name | Description |
---|---|
dataset - DatasetReference!
|
Dataset that owns the foreign keys |
Example
Query
mutation DeleteForeignKeys($dataset: DatasetReference!) {
deleteForeignKeys(dataset: $dataset) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
foreignKeys {
referencedDataset
links {
...RelationalLinkFragment
}
origin
}
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
quality {
originator
syntheticResult
trustScore
dashboardLink
checks {
...DataQualityCheckFragment
}
}
}
}
Variables
{"dataset": DatasetReference}
Response
{
"data": {
"deleteForeignKeys": {
"id": "4",
"type": "dataset",
"key": "xyz789",
"name": "abc123",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"foreignKeys": [ForeignKey],
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789",
"quality": DataQualityStatement
}
}
}
deleteForeignKeysV2
Description
Delete the foreign keys of a Dataset.
Foreign keys of Source
origin will be left untouched.
Response
Returns an UpdateDatasetPayload!
Arguments
Name | Description |
---|---|
input - DeleteForeignKeysInput!
|
List of input fields to delete foreign keys statement of a Dataset |
Example
Query
mutation DeleteForeignKeysV2($input: DeleteForeignKeysInput!) {
deleteForeignKeysV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
foreignKeys {
...ForeignKeyFragment
}
property
connection {
...ItemConnectionFragment
}
catalogCode
quality {
...DataQualityStatementFragment
}
}
}
}
Variables
{"input": DeleteForeignKeysInput}
Response
{
"data": {
"deleteForeignKeysV2": {
"clientMutationId": "abc123",
"item": Dataset
}
}
}
deleteItem
Description
Deletes an item. Available for Custom Items, Glossary Items, and Data Processes.
Response
Returns a DeleteItemPayload!
Arguments
Name | Description |
---|---|
input - DeleteItemInput
|
List of input fields to delete an item |
Example
Query
mutation DeleteItem($input: DeleteItemInput) {
deleteItem(input: $input) {
clientMutationId
}
}
Variables
{"input": DeleteItemInput}
Response
{
"data": {
"deleteItem": {
"clientMutationId": "abc123"
}
}
}
updateContact
Description
Updates a single contact.
Response
Returns an UpdateItemPayload!
Arguments
Name | Description |
---|---|
input - UpdateContactInput!
|
List of input fields to update a contact |
Example
Query
mutation UpdateContact($input: UpdateContactInput!) {
updateContact(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
}
}
Variables
{
"input": {
"clientMutationId": "xyz789",
"ref": "psmith@nowhere.far",
"email": "jdoe@nowhere.far",
"firstName": "John",
"lastName": "Doe",
"phone": "+1-202-555-0199",
"connections": [
{
"command": "MERGE",
"connectionRef": "curator",
"itemRefs": ["DATABASE/schema/table_name"]
}
]
}
}
Response
{
"data": {
"updateContact": {
"clientMutationId": "xyz789",
"item": Item
}
}
}
updateDataProcessOperations
Description
Update operations list on Data Process.
Response
Returns a DataProcess!
Arguments
Name | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Data Process |
operations - [DataProcessOperationInput!]!
|
List of inputs that state the Data Process operations |
Example
Query
mutation UpdateDataProcessOperations(
$ref: ItemReference!,
$operations: [DataProcessOperationInput!]!
) {
updateDataProcessOperations(
ref: $ref,
operations: $operations
) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
operations {
description {
...RichTextFragment
}
inputFields {
...FieldOperationFragment
}
outputFields {
...FieldOperationFragment
}
}
}
}
Variables
{
"ref": "DATABASE/schema/table_name",
"operations": [DataProcessOperationInput]
}
Response
{
"data": {
"updateDataProcessOperations": {
"id": 4,
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "abc123",
"operations": [DataProcessOperation]
}
}
}
updateDataProcessOperationsV2
Description
Update operations list on Data Process.
Response
Returns an UpdateDataProcessPayload!
Arguments
Name | Description |
---|---|
input - UpdateDataProcessOperationsInput!
|
List of input fields to update operations of a Data Process |
Example
Query
mutation UpdateDataProcessOperationsV2($input: UpdateDataProcessOperationsInput!) {
updateDataProcessOperationsV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
operations {
...DataProcessOperationFragment
}
}
}
}
Variables
{"input": UpdateDataProcessOperationsInput}
Response
{
"data": {
"updateDataProcessOperationsV2": {
"clientMutationId": "xyz789",
"item": DataProcess
}
}
}
updateDataQualityStatement
Description
Sets or updates Data Quality information of a Dataset.
Response
Returns a Dataset!
Arguments
Name | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Dataset |
quality - DataQualityStatementInput!
|
List of fields that state the data quality of the Dataset |
Example
Query
mutation UpdateDataQualityStatement(
$ref: ItemReference!,
$quality: DataQualityStatementInput!
) {
updateDataQualityStatement(
ref: $ref,
quality: $quality
) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
foreignKeys {
referencedDataset
links {
...RelationalLinkFragment
}
origin
}
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
quality {
originator
syntheticResult
trustScore
dashboardLink
checks {
...DataQualityCheckFragment
}
}
}
}
Variables
{
"ref": "DATABASE/schema/table_name",
"quality": DataQualityStatementInput
}
Response
{
"data": {
"updateDataQualityStatement": {
"id": 4,
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"foreignKeys": [ForeignKey],
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789",
"quality": DataQualityStatement
}
}
}
updateDataQualityStatementV2
Description
Sets or updates Data Quality information of a Dataset.
Response
Returns an UpdateDatasetPayload!
Arguments
Name | Description |
---|---|
input - UpdateDataQualityStatementInput!
|
List of input fields to update data quality statement of a Dataset |
Example
Query
mutation UpdateDataQualityStatementV2($input: UpdateDataQualityStatementInput!) {
updateDataQualityStatementV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
foreignKeys {
...ForeignKeyFragment
}
property
connection {
...ItemConnectionFragment
}
catalogCode
quality {
...DataQualityStatementFragment
}
}
}
}
Variables
{"input": UpdateDataQualityStatementInput}
Response
{
"data": {
"updateDataQualityStatementV2": {
"clientMutationId": "xyz789",
"item": Dataset
}
}
}
updateForeignKeys
Description
Replace the Api
foreign keys of a Dataset.
Response
Returns a Dataset!
Arguments
Name | Description |
---|---|
dataset - DatasetReference!
|
Dataset that owns the foreign keys |
foreignKeys - [ForeignKeyInput!]
|
List of the Api foreign keys |
Example
Query
mutation UpdateForeignKeys(
$dataset: DatasetReference!,
$foreignKeys: [ForeignKeyInput!]
) {
updateForeignKeys(
dataset: $dataset,
foreignKeys: $foreignKeys
) {
id
type
key
name
completion
description {
content
}
descriptionV2 {
content {
...TextFragment
}
summary
lifecycle
}
lastCatalogMetadataUpdate
foreignKeys {
referencedDataset
links {
...RelationalLinkFragment
}
origin
}
property
connection {
nodes {
...ItemFragment
}
edges {
...ItemEdgeFragment
}
pageInfo {
...PageInfoFragment
}
totalCount
}
catalogCode
quality {
originator
syntheticResult
trustScore
dashboardLink
checks {
...DataQualityCheckFragment
}
}
}
}
Variables
{
"dataset": DatasetReference,
"foreignKeys": [ForeignKeyInput]
}
Response
{
"data": {
"updateForeignKeys": {
"id": "4",
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"foreignKeys": [ForeignKey],
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "abc123",
"quality": DataQualityStatement
}
}
}
updateForeignKeysV2
Description
Replace the Api
foreign keys of a Dataset.
Response
Returns an UpdateDatasetPayload!
Arguments
Name | Description |
---|---|
input - UpdateForeignKeysInput!
|
List of input fields to update foreign keys of a Dataset |
Example
Query
mutation UpdateForeignKeysV2($input: UpdateForeignKeysInput!) {
updateForeignKeysV2(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
foreignKeys {
...ForeignKeyFragment
}
property
connection {
...ItemConnectionFragment
}
catalogCode
quality {
...DataQualityStatementFragment
}
}
}
}
Variables
{"input": UpdateForeignKeysInput}
Response
{
"data": {
"updateForeignKeysV2": {
"clientMutationId": "abc123",
"item": Dataset
}
}
}
updateItem
Description
Updates a single item (all types EXCEPT contact and datasource).
Response
Returns an UpdateItemPayload!
Arguments
Name | Description |
---|---|
input - UpdateItemInput!
|
List of input fields to update an item |
Example
Query
mutation UpdateItem($input: UpdateItemInput!) {
updateItem(input: $input) {
clientMutationId
item {
id
type
key
name
completion
description {
...RichTextFragment
}
descriptionV2 {
...ItemDescriptionFragment
}
lastCatalogMetadataUpdate
property
connection {
...ItemConnectionFragment
}
catalogCode
}
}
}
Variables
{"input": UpdateItemInput}
Response
{
"data": {
"updateItem": {
"clientMutationId": "xyz789",
"item": Item
}
}
}
Types
Boolean
Description
The Boolean
scalar type represents true
or false
.
CatalogPredicateInput
Description
Defines a condition on a String.
Fields
Input Field | Description |
---|---|
is - CatalogReference
|
Exact match between the tested Catalog Reference and the provided value |
in - [CatalogReference!]
|
Checks if the Catalog Reference is included into the provided list. |
Example
{
"is": CatalogReference,
"in": [CatalogReference]
}
CatalogReference
Description
Represents a reference to a catalog, either a user-defined code or internal id.
Example
CatalogReference
Command
Description
Used in update mutation to indicate how the provided value(s) must be processed.
Values
Enum Value | Description |
---|---|
|
Replace the former value(s) with the provided one |
|
For multivalued properties, and connection, merges the provided value(s) to the existing ones |
|
Remove the provided value(s) from the existing values |
|
Clear all the values of a property or empties a connection |
Example
"REPLACE"
ConnectionCreationInput
Description
Input type used to provide connected items for a new item
Fields
Input Field | Description |
---|---|
connectionRef - ConnectionReference!
|
Reference of the connection to feed (see naming conventions] |
itemRefs - [ItemReference]!
|
List of the items to connect through the connection |
Example
{
"connectionRef": "fields",
"itemRefs": ["DATABASE/schema/table_name"]
}
ConnectionFilterInput
Description
Defines a filter on a connection towards other items.
Fields
Input Field | Description |
---|---|
nodeTypes - [ItemType]
|
Allows retrieving specific item types when the connection concerns multiple ones |
Example
{"nodeTypes": ["dataset"]}
ConnectionMutationInput
Description
"Input type used to provide an update command on a specific connection"
Fields
Input Field | Description |
---|---|
command - Command!
|
The command drives how the provided values will be treated |
connectionRef - ConnectionReference!
|
Reference of the connection to update (see naming conventions] |
itemRefs - [ItemReference]
|
List of the references of the items to consider. Items must be of a type supported by the connection. For the CLEAR command, this is optional. Mandatory for all other commands. |
Example
{
"command": "REPLACE",
"connectionRef": "fields",
"itemRefs": ["DATABASE/schema/table_name"]
}
ConnectionPredicateInput
Description
Defines a condition on a connection towards other items.
Fields
Input Field | Description |
---|---|
ref - ConnectionReference
|
Reference of the target connection |
types - [ItemType]
|
Allows retrieving specific item types when the connection concerns multiple ones |
isEmpty - Boolean
|
Checks if at least one item is on the other end of the connection |
Example
{
"ref": "fields",
"types": ["dataset"],
"isEmpty": false
}
ConnectionReference
Description
Represents the reference of an outbound connection from an item as a String.
To learn more about connections and connection names, see the principles section and the naming convention section.
Example
"fields"
ContentType
Description
Item description content type.
Values
Enum Value | Description |
---|---|
|
|
|
Example
"RAW"
CreateContactInput
Description
Input type for creating a new item
Fields
Input Field | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
email - String
|
The email of the Contact |
phone - String
|
The phone of the Contact |
firstName - String!
|
The first name of the Contact (mandatory) |
lastName - String!
|
The last name of the Contact (mandatory) |
connections - [ConnectionCreationInput]
|
Links to other items |
Example
{
"clientMutationId": "abc123",
"email": "xyz789",
"phone": "abc123",
"firstName": "abc123",
"lastName": "xyz789",
"connections": [ConnectionCreationInput]
}
CreateItemInput
Description
Input type for creating a new item
Fields
Input Field | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
type - ItemType!
|
The type of the Item (mandatory) |
key - String
|
The key of the Item (will be generated if not provided) |
name - String!
|
The name of the Item (mandatory) |
description - RichTextInput
|
The description of the Item Use descriptionV3 instead. |
descriptionV2 - ItemDescriptionInput
|
The description of the Item with its content type Use descriptionV3 instead. |
descriptionV3 - ItemDescriptionInputV3
|
The description of the Item with its content type and its summary |
properties - [PropertyCreationInput]
|
Values of the properties of the newly created item |
connections - [ConnectionCreationInput]
|
Links to other items |
catalogCode - String
|
The catalog's code where the Item should be created |
Example
{
"clientMutationId": "abc123",
"type": "data-process",
"key": "abc123",
"name": "abc123",
"description": {"content": "abc123"},
"properties": [{"ref": "domain", "value": ["Supply chain"]}],
"connections": [
{"connectionRef": "inputs", "itemRefs": ["DATABASE/schema/table_name"]}
]
}
CreateItemPayload
Description
Result payload of the create item mutation
Example
{
"clientMutationId": "abc123",
"item": Item
}
CreateItemPayloadV2
Description
Result payload of the create item mutation
Example
{
"clientMutationId": "xyz789",
"item": Item
}
DataProcess
Description
A Data Process from the catalog
Fields
Field Name | Description |
---|---|
id - ID!
|
The internal identifier of the Data Process |
type - ItemType!
|
The type of the Data Process ('data-process') |
key - String!
|
The key of the Data Process |
name - String!
|
The name of the Data Process |
completion - Percentage
|
The completion level |
description - RichText
|
The description of the Data Process Use descriptionV2 instead. |
descriptionV2 - ItemDescription
|
The new version of the description of the Item |
lastCatalogMetadataUpdate - DateTime
|
The last update time of the Data Process in the catalog |
property - PropertyValue
|
Property of the Data Process |
Arguments
|
|
connection - ItemConnection
|
Resolve a named outbound link from the Data Process to other items. |
Arguments
|
|
catalogCode - String
|
The code of the catalog to which the item is related |
operations - [DataProcessOperation!]!
|
The Data Process operations field to field |
Example
{
"id": 4,
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789",
"operations": [DataProcessOperation]
}
DataProcessOperation
Description
A field to field operation on a Data Process
Fields
Field Name | Description |
---|---|
description - RichText
|
Description of the Data Process operation |
inputFields - [FieldOperation!]!
|
List of fields used as input in the operation |
outputFields - [FieldOperation!]!
|
List of fields used as output in the operation |
Example
{
"description": RichText,
"inputFields": [FieldOperation],
"outputFields": [FieldOperation]
}
DataProcessOperationInput
Description
Input type for creating a Data Process operation.
Fields
Input Field | Description |
---|---|
description - RichTextInput
|
Description of the Data Process operation |
inputFieldKeys - [ItemKey!]!
|
Keys of the input fields in the Data Process operation |
outputFieldKeys - [ItemKey!]!
|
Keys of the output fields in the Data Process operation |
Example
{
"description": RichTextInput,
"inputFieldKeys": [ItemKey],
"outputFieldKeys": [ItemKey]
}
DataQualityCheck
Description
A quality check is a test performed by a Data Quality solution
Fields
Field Name | Description |
---|---|
name - String!
|
Name of the check as defined in the DQ solution |
family - String
|
Family of check (for instance 'Completeness', 'Integrity', 'Freshness', etc.) |
description - RichText
|
Description of the check as defined in the DQ solution |
result - DataQualityCheckResult!
|
Result of the check |
lastExecutionTime - DateTime
|
Last time the check was executed |
checkLink - Url
|
Link to the check details in the DQ dashboard (if available) |
fieldNames - [String!]
|
Field source names used in the check |
score - String
|
Quality check score |
Example
{
"name": "abc123",
"family": "xyz789",
"description": RichText,
"result": "PASS",
"lastExecutionTime": "2023-01-01T00:00:00.000Z",
"checkLink": "https://soda.com/checks/check",
"fieldNames": ["abc123"],
"score": "abc123"
}
DataQualityCheckInput
Description
"Input type used to provide connected items for a new item"
Fields
Input Field | Description |
---|---|
name - String!
|
Name of the DataQuality check |
family - String
|
Family of the DataQuality check |
description - RichTextInput
|
Description of the DataQuality check |
result - DataQualityCheckResult!
|
Result of the DataQuality check |
lastExecutionTime - DateTime
|
Last execution time of the DataQuality check |
checkLink - Url
|
Link to the actual DataQuality check |
fieldNames - [String!]
|
Field source names used in the check |
score - String
|
Quality check score |
Example
{
"name": "xyz789",
"family": "xyz789",
"description": RichTextInput,
"result": "PASS",
"lastExecutionTime": "2023-01-01T00:00:00.000Z",
"checkLink": "https://soda.com/checks/check",
"fieldNames": ["xyz789"],
"score": "xyz789"
}
DataQualityCheckResult
Description
Result of a data quality check.
Values
Enum Value | Description |
---|---|
|
Data quality check passed. |
|
Data quality check failed. |
|
Data quality check passed with warning(s). |
Example
"PASS"
DataQualitySource
Description
Source of the data quality statement attached to a Dataset Expected to be a String with the source name.
Example
DataQualitySource
DataQualityStatement
Description
A DataQualityStatement holds the quality information on a Dataset
Fields
Field Name | Description |
---|---|
originator - DataQualitySource
|
Name of the system that performed the checks (ie. Soda, MonteCarlo, etc.) |
syntheticResult - DataQualityCheckResult
|
Synthetic result computed by Zeenea |
trustScore - Percentage
|
Overall trust score provided by the DQ tool (if available) |
dashboardLink - Url
|
Link to the Dataset dashboard in the DataQuality tool |
checks - [DataQualityCheck!]
|
List of the checks performed |
Example
{
"originator": DataQualitySource,
"syntheticResult": "PASS",
"trustScore": 0.87,
"dashboardLink": "https://soda.com/checks/check",
"checks": [DataQualityCheck]
}
DataQualityStatementInput
Description
"Input type used to add a DataQuality statement to a Dataset"
Fields
Input Field | Description |
---|---|
originator - DataQualitySource
|
Name of the system that performed the checks (ie. Soda, MonteCarlo, etc.) |
trustScore - Percentage
|
Synthetic score provided by the DataQuality tool |
dashboardLink - Url
|
Link to the Dataset dashboard in the DataQuality tool |
checks - [DataQualityCheckInput!]
|
List of checks provided by the DataQuality tool |
Example
{
"originator": DataQualitySource,
"trustScore": 0.87,
"dashboardLink": "https://soda.com/checks/check",
"checks": [DataQualityCheckInput]
}
Dataset
Description
A Dataset from the catalog
Fields
Field Name | Description |
---|---|
id - ID!
|
The internal identifier of the Dataset |
type - ItemType!
|
The type of the Dataset ('dataset') |
key - String!
|
The key of the Dataset |
name - String!
|
The name of the Dataset |
completion - Percentage
|
The completion level |
description - RichText
|
The description of the Dataset Use descriptionV2 instead. |
descriptionV2 - ItemDescription
|
The new version of the description of the Item |
lastCatalogMetadataUpdate - DateTime
|
The last update time of the Dataset in the catalog |
foreignKeys - [ForeignKey!]
|
The foreign keys of the Dataset |
property - PropertyValue
|
Property of the Dataset |
Arguments
|
|
connection - ItemConnection
|
Resolve a named outbound link from the Dataset to other items. |
Arguments
|
|
catalogCode - String
|
The code of the catalog to which the item is related |
quality - DataQualityStatement
|
Quality statement |
Example
{
"id": 4,
"type": "dataset",
"key": "xyz789",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"foreignKeys": [ForeignKey],
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789",
"quality": DataQualityStatement
}
DatasetReference
Description
Represents a unique reference as a String to identify a Dataset.
Example
DatasetReference
DatePredicateInput
Description
Defines a condition on a DateTime.
Fields
Input Field | Description |
---|---|
is - DateTime
|
Exact match between the tested date and the provided value |
lt - DateTime
|
Checks lower than (before) the provided value |
lte - DateTime
|
Checks lower than (before) or equal to the provided value |
gt - DateTime
|
Checks greater than (after) the provided value |
gte - DateTime
|
Checks greater than (after) or equal to the provided value |
isEmpty - Boolean
|
isEmpty is TRUE (no value/empty) (==0) or isEmpty is FALSE (at least one value) (>0) |
Example
{
"is": "2023-01-01T00:00:00.000Z",
"lt": "2023-01-01T00:00:00.000Z",
"lte": "2023-01-01T00:00:00.000Z",
"gt": "2023-01-01T00:00:00.000Z",
"gte": "2023-01-01T00:00:00.000Z",
"isEmpty": false
}
DateTime
Description
Represents a date-time as a string in ISO 8601 format
Example
"2023-01-01T00:00:00.000Z"
DeleteContactInput
Description
Input type for deleting a Contact.
Fields
Input Field | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
ref - ItemReference!
|
Reference used to identify the Contact |
Example
{
"clientMutationId": "xyz789",
"ref": "DATABASE/schema/table_name"
}
DeleteDataProcessOperationsInput
Description
Input type for deleting operations on a Data Process.
Fields
Input Field | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Data Process |
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{
"ref": "DATABASE/schema/table_name",
"clientMutationId": "abc123"
}
DeleteDataQualityStatementInput
Description
Input type for deleting a data quality statement.
Fields
Input Field | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Dataset |
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{
"ref": "DATABASE/schema/table_name",
"clientMutationId": "abc123"
}
DeleteForeignKeysInput
Description
Input type for deleting foreign keys of a Dataset.
Fields
Input Field | Description |
---|---|
ref - DatasetReference!
|
Dataset that owns the foreign keys |
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{
"ref": DatasetReference,
"clientMutationId": "xyz789"
}
DeleteItemInput
Description
Input type for deleting an Item.
Fields
Input Field | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
ref - ItemReference!
|
Reference used to identify the Item |
Example
{
"clientMutationId": "xyz789",
"ref": "DATABASE/schema/table_name"
}
DeleteItemPayload
Description
Result payload of the delete item mutation
Fields
Field Name | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{"clientMutationId": "xyz789"}
DescriptionLifecycle
Description
Item Description lifecycle (Not yet implemented)
Values
Enum Value | Description |
---|---|
|
The current description is synchronized with the source description |
|
The current description is synchronized with the description defined in Zeenea |
Example
"SYNCED_WITH_SOURCE"
Field
Fields
Field Name | Description |
---|---|
id - ID!
|
The internal identifier of the Field |
type - ItemType!
|
The type of the Field ('field') |
key - String!
|
The key of the Field |
name - String!
|
The name of the Field |
completion - Percentage
|
The completion level |
description - RichText
|
The description of the Field Use descriptionV2 instead. |
descriptionV2 - ItemDescription
|
The new version of the description of the Item |
lastCatalogMetadataUpdate - DateTime
|
The last update time of the Field in the catalog |
property - PropertyValue
|
Property of the Field |
Arguments
|
|
connection - ItemConnection
|
Resolve a named outbound link from the Field to other items. |
Arguments
|
|
catalogCode - String
|
The code of the catalog to which the item is related |
fieldType - String!
|
The normalized type of the field |
fieldNativeType - String!
|
The native type of the field as defined in the source system |
canBeNull - Boolean!
|
Whether the field is nullable |
multivalued - Boolean!
|
Whether the field supports multiple values |
primaryKey - Boolean!
|
Whether the field is a primary key |
foreignKey - Boolean!
|
Whether the field is a foreign key |
businessKey - Boolean!
|
Whether the field is a business key |
Example
{
"id": 4,
"type": "dataset",
"key": "xyz789",
"name": "abc123",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789",
"fieldType": "xyz789",
"fieldNativeType": "abc123",
"canBeNull": false,
"multivalued": false,
"primaryKey": false,
"foreignKey": true,
"businessKey": false
}
FieldOperation
Description
Reference to a field used in a DataProcessOperation
Fields
Field Name | Description |
---|---|
ref - ItemReference!
|
Reference used to identify the field |
item - Item
|
The field used in the DataProcessOperation |
isValid - Boolean!
|
Whether the field is a valid item in the catalog or not |
Example
{
"ref": "DATABASE/schema/table_name",
"item": Item,
"isValid": false
}
ForeignKey
Fields
Field Name | Description |
---|---|
referencedDataset - String!
|
Reference of the referenced dataset |
links - [RelationalLink!]!
|
One or more (for compound keys) pairs of foreign key field/referenced field |
origin - ForeignKeyOrigin!
|
The origin of this foreign key, can be Api or Source |
Example
{
"referencedDataset": "abc123",
"links": [RelationalLink],
"origin": ForeignKeyOrigin
}
ForeignKeyInput
Fields
Input Field | Description |
---|---|
referencedDataset - ReferencedDatasetReference!
|
Reference of the referenced dataset |
links - [RelationalLinkInput!]!
|
One or more (for compound keys) pairs of foreign key field/referenced field |
Example
{
"referencedDataset": ReferencedDatasetReference,
"links": [RelationalLinkInput]
}
ForeignKeyOrigin
Description
Origin of the foreign key. Can be Api or Source.
Example
ForeignKeyOrigin
GenericItem
Description
A generic item from the catalog
Fields
Field Name | Description |
---|---|
id - ID!
|
The internal identifier of the item |
type - ItemType!
|
The type of the item |
key - String!
|
The key of the item |
name - String!
|
The name of the Item |
completion - Percentage
|
The completion level (null if not available on the given item) |
description - RichText
|
The description of the Item Use descriptionV2 instead. |
descriptionV2 - ItemDescription
|
The new version of the description of the Item |
lastCatalogMetadataUpdate - DateTime
|
The last update time of the item in the catalog |
property - PropertyValue
|
Property of the item |
Arguments
|
|
connection - ItemConnection
|
Resolve a named outbound link from the item to other items. |
Arguments
|
|
catalogCode - String
|
The code of the catalog to which the item is related |
Example
{
"id": "4",
"type": "dataset",
"key": "xyz789",
"name": "abc123",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "abc123"
}
ID
Description
The ID
scalar type represents a unique identifier, often used to refetch an object or as key for a cache. The ID type appears in a JSON response as a String; however, it is not intended to be human-readable. When expected as an input type, any string (such as "4"
) or integer (such as 4
) input value will be accepted as an ID.
Example
"4"
Int
Description
The Int
scalar type represents non-fractional signed whole numeric values. Int can represent values between -(2^31) and 2^31 - 1.
Example
123
Item
Description
Interface of all items, which is specialized for some item types
Fields
Field Name | Description |
---|---|
id - ID!
|
The internal identifier of the item |
type - ItemType!
|
The type of the item |
key - String!
|
The key of the item |
name - String!
|
The name of the item |
completion - Percentage
|
The completion level (null if not available on the given item) |
description - RichText
|
The description of the item Use descriptionV2 instead. |
descriptionV2 - ItemDescription
|
The new version of the description of the item |
lastCatalogMetadataUpdate - DateTime
|
The last update time of the item in the catalog |
property - PropertyValue
|
Property of the item |
Arguments
|
|
connection - ItemConnection
|
Resolve a named outbound link from the item to other items. |
Arguments
|
|
catalogCode - String
|
The code of the catalog to which the item is related |
Possible Types
Item Types |
---|
Example
{
"id": 4,
"type": "dataset",
"key": "abc123",
"name": "xyz789",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789"
}
ItemConnection
Description
A paginated collection of items following the Relay convention.
Fields
Field Name | Description |
---|---|
nodes - [Item!]!
|
Shortcut to the fetched page of items. Use it when you do not want to get cursor . Inspired by GitHub GraphQL API schema. |
edges - [ItemEdge]!
|
List of items embedded into an Edge object type to follow Relay conventions |
pageInfo - PageInfo!
|
Information about the current page. Necessary to fetch the next pages(s) |
totalCount - Int!
|
The total count of items in the collection |
Example
{
"nodes": [Item],
"edges": [ItemEdge],
"pageInfo": PageInfo,
"totalCount": 123
}
ItemDescription
Description
Item's description (Not yet implemented)
Fields
Field Name | Description |
---|---|
content - Text!
|
|
summary - LongText!
|
|
lifecycle - DescriptionLifecycle!
|
Example
{
"content": Text,
"summary": LongText,
"lifecycle": "SYNCED_WITH_SOURCE"
}
ItemDescriptionInput
Description
Input type for creating or updating an item description
Fields
Input Field | Description |
---|---|
lifecycle - DescriptionLifecycle
|
|
content - TextInput
|
Example
{"lifecycle": "SYNCED_WITH_SOURCE", "content": TextInput}
ItemDescriptionInputV3
Description
Input type for creating or updating an item description
Fields
Input Field | Description |
---|---|
lifecycle - DescriptionLifecycle
|
|
content - TextInput
|
|
summary - LongText
|
|
recomputeSummary - Boolean
|
Example
{
"lifecycle": "SYNCED_WITH_SOURCE",
"content": TextInput,
"summary": LongText,
"recomputeSummary": true
}
ItemEdge
ItemFilterInput
Description
Input type used to filter a request. A filter contains a list of predicates (conditions) that will be tested against the items to filter the results of the items query. Each filter must contain one and only one predicate amongst the possible ones. Multiple filters can be provided to the items query (filters are combined with a AND operator)
Fields
Input Field | Description |
---|---|
property - PropertyPredicateInput
|
Express a condition on a property |
catalogRef - CatalogPredicateInput
|
Express a condition on a catalog reference |
connection - ConnectionPredicateInput
|
Express a condition on one connection |
completion - NumberPredicateInput
|
Condition on the completion level |
name - StringPredicateInput
|
Condition on the name |
description - StringPredicateInput
|
Condition on the description |
lastCatalogMetadataUpdate - DatePredicateInput
|
Condition on the lastCatalogMetadataUpdate |
Example
{
"property": {"ref": "prop_code", "contains": ["Nice"]},
"catalogRef": CatalogPredicateInput,
"connection": ConnectionPredicateInput,
"completion": NumberPredicateInput,
"name": {"is": "Finance"},
"description": {"is": "Finance"},
"lastCatalogMetadataUpdate": DatePredicateInput
}
ItemKey
Description
Represents the unique key of the item (see Zeenea documentation for items key specifications).
Example
ItemKey
ItemMutationInput
Description
Input type representing all the updates to apply to an item
Fields
Input Field | Description |
---|---|
key - String
|
New key of the item |
name - String
|
New name of the item |
description - RichTextInput
|
Description of the Item: use an empty content to remove description Use descriptionV3 instead. |
descriptionV2 - ItemDescriptionInput
|
The description of the Item with its content type Use descriptionV3 instead. |
descriptionV3 - ItemDescriptionInputV3
|
The description of the Item with its content type and its summary |
properties - [PropertyMutationInput]
|
List of the updates to apply to the item properties, using a command pattern |
connections - [ConnectionMutationInput]
|
List of the updates to apply to the item connections, using a command pattern |
Example
{
"key": "xyz789",
"name": "abc123",
"description": RichTextInput,
"descriptionV2": ItemDescriptionInput,
"descriptionV3": ItemDescriptionInputV3,
"properties": [PropertyMutationInput],
"connections": [ConnectionMutationInput]
}
ItemReference
Description
Represents a unique reference as a String to identify an item.
The reference value can be:
- the technical id of the item, usually only available from a former call to the API
- the unique key of the item (see Zeenea documentation for items key specifications). For items of type "contact", the reference is the email address.
The lookup strategy tries to locate the item in the above order.
If the item is not found or more that one items match, an error is raised.
Technical ids and keys are unique in Zeenea, so they are sufficient to find the item. If you want to use names instead, you might have to use the itemByName query method, as name uniqueness is not enforced.
Example
"DATABASE/schema/table_name"
ItemType
Description
The type of an Item in a String format.
Valid values:
Item type | Value |
---|---|
Dataset | dataset |
Field | field |
Visualization | visualization |
Data process | data-process |
Contact | contact |
Datasource | datasource |
Category | category |
Custom Item Type | Code of the custom item as defined in Zeenea metamodel |
Glossary Item Type | Code of the glossary item as defined in Zeenea metamodel |
Example
"dataset"
LongText
Description
Represents a Long Text as a string (Not implemented yet).
Example
LongText
Node
Description
An object with a Globally Unique ID
Fields
Field Name | Description |
---|---|
id - ID!
|
The ID of the object. |
Possible Types
Node Types |
---|
Example
{"id": 4}
Number
Description
Represents any number
Example
0.2
NumberPredicateInput
Description
Defines a condition on a Number.
Fields
Input Field | Description |
---|---|
is - Number
|
Exact match between the tested number and the provided value |
lt - Number
|
Checks lower than the provided value |
lte - Number
|
Checks lower than or equal to the provided value |
gt - Number
|
Checks greater than the provided value |
gte - Number
|
Checks greater than or equal to the provided value |
isEmpty - Boolean
|
isEmpty is TRUE (no value/empty) (==0) or isEmpty is FALSE (at least one value) (>0) |
Example
{"is": 0.2, "lt": 0.2, "lte": 0.2, "gt": 0.2, "gte": 0.2, "isEmpty": false}
PageInfo
Description
Information about the current page of a Connection, following Relay conventions. Used to fetch the next/previous page if available.
Fields
Field Name | Description |
---|---|
startCursor - String
|
Cursor of the first item in the page (used as 'before' parameter of the connection to fetch the previous page) |
hasNextPage - Boolean!
|
Whether there is a next page |
hasPreviousPage - Boolean!
|
Whether there is a previous page |
endCursor - String
|
Cursor of the last item in the page (used as 'after' parameter of the connection to fetch the next page) |
Example
{
"startCursor": "xyz789",
"hasNextPage": true,
"hasPreviousPage": true,
"endCursor": "xyz789"
}
Percentage
Description
Represents a percentage in base 1 (values range from 0.0 to 1.0)
Example
0.87
PropertyCreationInput
Description
Input type used to provide property values for a new item
Fields
Input Field | Description |
---|---|
ref - PropertyReference!
|
Reference of the property to feed |
value - PropertyValue!
|
Value of the property (as an array of strings) |
Example
{
"ref": PropertyReference,
"value": ["Value 1", "Value 2"]
}
PropertyMutationInput
Description
Input type used to provide an update command on a specific property
Fields
Input Field | Description |
---|---|
command - Command!
|
The command drives how the provided values will be treated |
ref - PropertyReference!
|
Reference of the property to update (see naming conventions] |
value - PropertyValue
|
List of the values to consider (as an array of strings). For the CLEAR command, this is optional. Mandatory for all other commands. |
Example
{
"command": "REPLACE",
"ref": PropertyReference,
"value": ["Value 1", "Value 2"]
}
PropertyPredicateInput
Description
Defines a condition on a property value. Valid predicates must carry a mandatory property reference (to identify the target property), and one and only one "test" attribute, that will be evaluated against the property value.
Fields
Input Field | Description |
---|---|
ref - PropertyReference!
|
Reference of the target property |
is - PropertyValue
|
Exactly matches the provided property value. For multi-valued property, both lists must contain exactly the same values (the order is not taken into account) |
in - PropertyValue
|
Checks if the property value is included into the provided list. For multi-valued properties, all the values must be present in the provided list. |
lt - PropertyValue
|
Checks if the property value is lower than the provided value. Only works for single-value properties of type Number, Date or DateTime |
lte - PropertyValue
|
Checks if the property value is lower than of equal to the provided value. Only works for single-value properties of type Number, Date or DateTime |
gt - PropertyValue
|
Checks if the property value is greater than to the provided value. Only works for single-value properties of type Number, Date or DateTime |
gte - PropertyValue
|
Checks if the property value is greater than of equal to the provided value. Only works for single-value properties of type Number, Date or DateTime |
contains - PropertyValue
|
Checks if the property value contains the provided value. Only works for multi-valued properties |
startsWith - PropertyValue
|
Starts with match between the tested PropertyValue and the provided value. Only works for sourceName and sourceDescription |
endsWith - PropertyValue
|
Ends with match between the tested PropertyValue and the provided value. Only works for sourceName and sourceDescription |
isEmpty - Boolean
|
Checks if the property is valued (at least one value is set for multi-valued properties) |
Example
{"ref": "prop_code", "contains": ["Nice"]}
PropertyReference
Description
Represents a named reference to a property as defined in the metamodel.
The reference of the property will be resolved in the following order:
- code of the property
- name of the property (case sensitive)
To learn more about how to retrieve item properties, see the principles section and the naming convention section.
Example
PropertyReference
PropertyValue
Description
Represents the value of a property. As properties can have multiple values, it is mapped to an array of strings. For single-value properties, the table will only contain one value. An empty table means the property is not set.
Example
["Value 1", "Value 2"]
ReferencedDatasetReference
Description
Represents a unique reference as a String to identify the Dataset referenced by a foreign key. To reference this Dataset you can use either its:
- id
- key
- path
- source name
- name
This referenced Dataset must belong to the same Datasource as the Dataset that owns the foreign key.
Example
ReferencedDatasetReference
RelationalLink
RelationalLinkInput
Example
{
"foreignKeyFieldName": "abc123",
"referencedFieldName": "abc123"
}
RichText
Description
Item's description
Fields
Field Name | Description |
---|---|
content - String!
|
Content of the Item's description |
Example
{"content": "abc123"}
RichTextInput
Description
Input type for creating or updating a Rich Text.
Fields
Input Field | Description |
---|---|
content - String!
|
Content of the Rich Text (mandatory) |
Example
{"content": "xyz789"}
SemanticItem
Description
A Semantic Item from the catalog
Fields
Field Name | Description |
---|---|
id - ID!
|
The internal identifier of the item |
type - ItemType!
|
The type of the item |
key - String!
|
The key of the item |
name - String!
|
The name of the Item |
completion - Percentage
|
The completion level (null if not available on the given item) |
description - RichText
|
The description of the Item Use descriptionV2 instead. |
descriptionV2 - ItemDescription
|
The new version of the description of the Item |
lastCatalogMetadataUpdate - DateTime
|
The last update time of the item in the catalog |
alternativeNames - [String!]!
|
The alternative names of the Semantic Item |
property - PropertyValue
|
Property of the item |
Arguments
|
|
connection - ItemConnection
|
Resolve a named outbound link from the item to other items. |
Arguments
|
|
catalogCode - String
|
The code of the catalog to which the item is related |
Example
{
"id": 4,
"type": "dataset",
"key": "xyz789",
"name": "abc123",
"completion": 0.87,
"description": RichText,
"descriptionV2": ItemDescription,
"lastCatalogMetadataUpdate": "2023-01-01T00:00:00.000Z",
"alternativeNames": ["abc123"],
"property": ["Value 1", "Value 2"],
"connection": ItemConnection,
"catalogCode": "xyz789"
}
String
Description
The String
scalar type represents textual data, represented as UTF-8 character sequences. The String type is most often used by GraphQL to represent free-form human-readable text.
Example
"xyz789"
StringPredicateInput
Description
Defines a condition on a String.
Fields
Input Field | Description |
---|---|
is - String
|
Exact match between the tested string and the provided value |
startsWith - String
|
Starts with match between the tested string and the provided value |
endsWith - String
|
Ends with match between the tested string and the provided value |
isEmpty - Boolean
|
isEmpty is TRUE (no value/empty) (==0) or isEmpty is FALSE (at least one value) (>0) |
Example
{"is": "Finance"}
Text
Description
Text content
Fields
Field Name | Description |
---|---|
content - String!
|
Content of the Item's description |
contentType - ContentType!
|
Example
{"content": "abc123", "contentType": "RAW"}
TextInput
Description
Input type for creating or updating a text content (Not yet implemented)
Fields
Input Field | Description |
---|---|
content - String!
|
|
contentType - ContentType!
|
Example
{"content": "xyz789", "contentType": "RAW"}
UpdateContactInput
Description
Input type for updating an existing item
Fields
Input Field | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
ref - ItemReference!
|
The ref of the Item (mandatory) |
email - String
|
The key of the Item (will be generated if not provided) |
firstName - String
|
The first name of the Contact |
lastName - String
|
The last name of the Contact |
phone - String
|
The phone of the Contact |
connections - [ConnectionMutationInput]
|
List of the updates to apply to the contact connections, using a command pattern |
Example
{
"clientMutationId": "xyz789",
"ref": "psmith@nowhere.far",
"email": "jdoe@nowhere.far",
"firstName": "John",
"lastName": "Doe",
"phone": "+1-202-555-0199",
"connections": [
{
"command": "MERGE",
"connectionRef": "curator",
"itemRefs": ["DATABASE/schema/table_name"]
}
]
}
UpdateDataProcessOperationsInput
Description
Input type for updating operations on a Data Process
Fields
Input Field | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Data Process |
operations - [DataProcessOperationInput!]!
|
List of inputs that state the Data Process operations |
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{
"ref": "DATABASE/schema/table_name",
"operations": [DataProcessOperationInput],
"clientMutationId": "abc123"
}
UpdateDataProcessPayload
Description
Result payload of the data process mutations
Fields
Field Name | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
item - DataProcess
|
The updated data process |
Example
{
"clientMutationId": "xyz789",
"item": DataProcess
}
UpdateDataQualityStatementInput
Description
Input type for updating a data quality statement
Fields
Input Field | Description |
---|---|
ref - ItemReference!
|
Unique reference of a Dataset |
quality - DataQualityStatementInput!
|
List of fields that state the data quality of the Dataset |
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{
"ref": "DATABASE/schema/table_name",
"quality": DataQualityStatementInput,
"clientMutationId": "xyz789"
}
UpdateDatasetPayload
Description
Result payload of the dataset mutations
Example
{
"clientMutationId": "abc123",
"item": Dataset
}
UpdateForeignKeysInput
Description
Input type for updating foreign keys of a Dataset
Fields
Input Field | Description |
---|---|
ref - DatasetReference!
|
Dataset that owns the foreign keys |
foreignKeys - [ForeignKeyInput!]
|
List of the Api foreign keys |
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
Example
{
"ref": DatasetReference,
"foreignKeys": [ForeignKeyInput],
"clientMutationId": "abc123"
}
UpdateItemInput
Description
Input type for updating an existing item
Fields
Input Field | Description |
---|---|
clientMutationId - String
|
An optional mutation ID that will be sent back in the payload if provided in the mutation call to correlate the response with the query |
ref - ItemReference!
|
The ref of the Item (mandatory) |
updates - ItemMutationInput!
|
All the updates to apply to the target item |
Example
{
"clientMutationId": "abc123",
"ref": "DATABASE/schema/table_name",
"updates": ItemMutationInput
}
UpdateItemPayload
Description
Result payload of the update item mutation
Example
{
"clientMutationId": "abc123",
"item": Item
}
Url
Description
A well formed URL as a String
Example
"https://soda.com/checks/check"